Classes in Java (Java Class) are templates for creating objects, in what is known as object-oriented programming, which is one of the main software development paradigms today.
If we look for Java in Wikipedia, in the definition that is available for the programming language with this name we will find:
Java is a general-purpose, concurrent, object-oriented programming language that was specifically designed to have as few implementation dependencies as possible.
There is something that stands out from this short definition: object-oriented. What does it mean?
According to Wikipedia:
Object Oriented Programming or OOP (OOP) is a programming paradigm that uses objects in their interactions to design applications and computer programs. It is based on various techniques, including inheritance, cohesion, abstraction, polymorphism, coupling, and encapsulation. Its use became popular in the early 1990s. Today, there is a wide variety of programming languages that support object orientation.
I am going to define them in a simpler way: it is the best programming paradigm created by man. It allows you to write code in an orderly way, reuse it, order the information and do a thousand and one tasks that without this paradigm would be unthinkable. Almost all current programming languages have this kind of paradigm, but in my opinion, Java is synonymous with object-oriented language. It is one of its main characteristics.
What I will try to do now is explain what a class is while giving you some tips when programming in Java. We have to be educated in the way we program and keep an order in what we do.
What is a class?
Classes in Java are basically a template used to create an object. If we imagine classes in the world we live in, we could say that the class “person” is a template for how a human being should be. Each and every one of us human beings are objects of the class “person”, since we are all persons. The class “person” contains the definition of a human being, while each human being is an instance or object of that class.
In order to understand this we must see it with examples. We are going to create a project in Netbeans that I am going to call JavaExamples.
We see that a file named JavaExamples.java (Java File Class) is created. This is the main class, the one that contains the public static void main method. When running a program, whatever is set in the void main will be the first thing to run. The JavaExamples class is an example of a class, but it is not clear what concepts I would like to convey to you, so we are going to create a class that we will call “persona“.
Now we are going to start building the class “person”. We need to set the properties of the persona class. As people, what properties do we have?
We could talk about a name, surname, a date we were born, our age and gender. There are many other properties that we could mention, but for now we will only take into account the ones that we have listed. They are simply characteristics that every human being possesses, at least in most cases.
To establish these properties within the class we create variables, taking care to assign each property the type of data that corresponds to it. That said, we declare the properties of the persona class:
1 2 3 4 5 |
private String name; private String lastName; private String birthDate; private int age; private String gender; |
By setting the properties as private we are ensuring that within the person class these properties can be accessed and manipulated, but not from another class, unless the user so decides and it is carried out through public methods. We will see this a little later.
Once the properties of the class are established, we create a constructor. The constructor is a method with the same name as the class itself, where the parameters and actions to be executed are established whenever a new object or instance is created (object and instance are basically the same). In Java the so-called “functions” of other languages are known as “methods“. Our constructor would be:
1 2 3 4 5 6 7 |
public persona(String nombre, String apellido, String fecha, int edad, String genero) { name = nombre; lastName = apellido; birthDate = fecha; age = edad; gender = genero; } |
When we are going to build an object of the class person, we will be forced to establish a series of initial parameters. When the instance is created, the first task the class will perform is to assign the constructor parameters to the class properties. These properties, as we already mentioned, have private access within the class and cannot be modified from the outside. However, we can grant you restricted access from outer classes to the properties of our “person” class. For this we establish the so-called getters and setters that are methods to obtain or modify properties of a class.
An example would be the setter and getter for the Name property.
1 2 3 4 5 6 7 |
public void setName(String nombre) { name = nombre; } public String getName() { return name; } |
When we invoke the setter setName(String nombre) we are changing the name property for the parameter that we introduce, that is, nombre (name in Spanish). This method is of type void, so it does not return any value. Just change the name property and nothing else.
When we invoke the getter getName() we will “call” the name property and obtain its value. The method is type String, so it returns a string of characters with the value of the name property, hence the return name command is used.
Now we set the getters and setters for all the properties. Our person class would look like this:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
public class persona { //Propiedades private String name; private String lastName; private String birthDate; private int age; private String gender; //Constructor public persona(String nombre, String apellido, String fecha, int edad, String genero) { name = nombre; lastName = apellido; birthDate = fecha; age = edad; gender = genero; } //Métodos - getters&setters public void setName(String nombre) { name = nombre; } public String getName() { return name; } public void setLastName(String apellido) { lastName = apellido; } public String getLastName() { return lastName; } public void setBirthDate(String fecha) { birthDate = fecha; } public String getBirthDate() { return birthDate; } public void setAge(int edad) { age = edad; } public int getAge() { return age; } public void setGender(String genero) { gender = genero; } public String getGender() { return gender; } } |
So the internal structure of our “person” class should look like this:
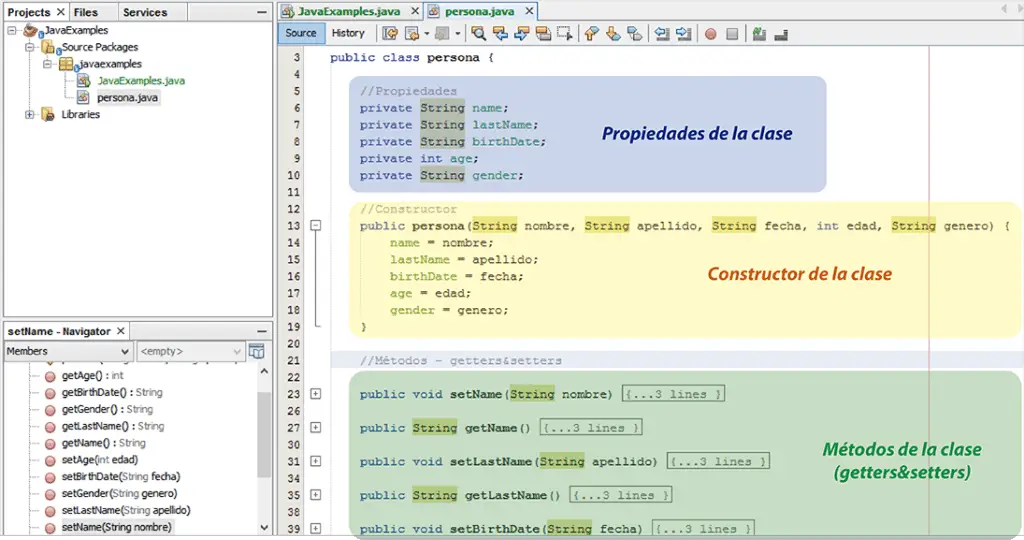
Our “people” class is ready. We have created a template to “create” people. But how can we accomplish this? We go to our main class and create an instance of the person class.
1 |
persona p1 = new persona("Antony", "García González", "30/7/1992", 22, "M"); |
This new person that I have created with the name of p1 has a name “Antony”, surnames “García González”, date of birth “7/30/1992”, age 22 and is male.
The object p1 represents me. I am Antony García González, but within Java I am an object named p1. We create this object following the following syntax:
Class_name object_name = new Class_name(constructor parameters).
Classes in Java are always instantiated in the same way. To instantiate is to create an object of a class. So this object that we created, within our application is called p1 but for the software user it has my name, Antony.
From the object p1 we can “obtain” its properties or “modify” them. If we write in our code in the Netbeans IDE “p1.” The following will appear:
We see that the Netbeans wizard presents us with all the methods available for the p1 object. That is the advantage of classes in Java, that a single object contains a large number of methods inside it to carry out different procedures summarized in a single line of code. For now we have worked with short sentences, just setting properties or getting their values. But we could well add a method that does something much more complicated and extensive and invoke it after having created an object of the class in question.
There are some methods that we did not set, such as equals, hashCode, etc … These are methods inherited from the Object class. We will study the concept of inheritance in other contributions. For now, it is enough to know that we have at our disposal the getters and setters that we established when building our “persona” class.
We can build as many objects as we want.
We can suddenly change some name of some object.
We can test the getters by printing the information from the p2 object to the console.
By using getters, we simply get character strings with the values of the properties of the set. We print these strings to the console with System.out.println and at the bottom of the window we see the results. In the first impression the name and other properties are those that we established in the creation of the object p2. Then with the setters we change some properties and when we print again we see that the information has changed.
I hope the concept of classes in Java, their construction and the creation of objects has been clear. In the next few days I will be posting a little more about the thousand and one things we can achieve with object-oriented programming. See ya’.